이번 포스트에서는 자바스크립트 함수 포스트에서 다루지 못했던 화살표 함수 특징에 대해서 정리해 보았습니다
화살표 함수의 선언 방법들
괄호는 생략 가능합니다.
하지만 매개변수가 하나의 단순한 형태의 경우에만 생략이 가능합니다.
() => expression
param => expression
(param) => expression
(param1, paramN) => expression
() => {
statements
}
param => {
statements
}
(param1, paramN) => {
statements
}
const func = (param1) => param1 * param1;
const func = (param1, param2) => {
return param1 + param2;
};
화살표 함수의 특징
this, arguments, super에 바인딩 되지 않으므로 메소드로 사용하면 안됩니다.
생성자로 사용하면 안됩니다. new로 호출하면 TypeError를 던집니다.
화살표 함수는 yield 문을 사용할 수 없습니다.
화살표 함수를 이용한 익명 함수
기존의 익명 함수와 화살표 함수의 익명 함수를 비교 정리하였습니다.
// Traditional anonymous function
(function (a) {
return a + 100;
});
// 1. Remove the word "function" and place arrow between the argument and opening body brace
(a) => {
return a + 100;
};
// 2. Remove the body braces and word "return" — the return is implied.
(a) => a + 100;
// 3. Remove the parameter parentheses
a => a + 100;
화살표 함수의 body
expression body와 block body 형태로 사용할 수 있습니다.
expression body는 한 줄로 명시하는 방식입니다. 이때는 return을 표기하지 않아도 됩니다.
block body 형태는 return을 반드시 명시해줘야 합니다.
const func = (x) => x * x;
// expression body syntax, implied "return"
const func2 = (x, y) => {
return x + y;
};
// with block body, explicit "return" needed
화살표 함수에서 객체 반환
화살표 함수에서 {} 중괄호는 body로 인식하기 때문에 아래와 같이 반환시 오류가 발생합니다.
const func = () => { foo: 1 };
// Calling func() returns undefined!
const func2 = () => { foo: function () {} };
// SyntaxError: function statement requires a name
const func3 = () => { foo() {} };
// SyntaxError: Unexpected token '{'
객체를 반환하기 위해서는 소괄호()로 감싸줘야 합니다.
const func = () => ({ foo: 1 });
메소드로 사용 금지
this를 가지지 않기 때문에 메소드로 사용하면 안됩니다.
"use strict";
const obj = {
i: 10,
b: () => console.log(this.i, this),
c() {
console.log(this.i, this);
},
};
obj.b(); // logs undefined, Window { /* … */ } (or the global object)
obj.c(); // logs 10, Object { /* … */ }
argument binding
화살표 함수(arrow functions)는 자체적인 arguments 객체를 가지고 있지 않습니다.
const f1 = () => arguments[0];
console.log(f1(10)); // {}
대신 rest parameter를 통해 인자를 받을 수 있습니다.
const f2 = (...args) => args[0];
console.log(f2(10)); // 10
또한 화살표 함수는 해당 화살표 함수가 포함된 주변 스코프의 arguments 객체를 참조할 수 있습니다.
function foo(n) {
const f = () => arguments[0] + n; // foo's implicit arguments binding. arguments[0] is n
return f();
}
foo(3); // 3 + 3 = 6
화살표 함수의 사용 예제
// An empty arrow function returns undefined
const empty = () => {};
(() => "foobar")();
// Returns "foobar"
// (this is an Immediately Invoked Function Expression)
const simple = (a) => (a > 15 ? 15 : a);
simple(16); // 15
simple(10); // 10
const max = (a, b) => (a > b ? a : b);
// Easy array filtering, mapping, etc.
const arr = [5, 6, 13, 0, 1, 18, 23];
const sum = arr.reduce((a, b) => a + b);
// 66
const even = arr.filter((v) => v % 2 === 0);
// [6, 0, 18]
const double = arr.map((v) => v * 2);
// [10, 12, 26, 0, 2, 36, 46]
// More concise promise chains
promise
.then((a) => {
// …
})
.then((b) => {
// …
});
// Parameterless arrow functions that are visually easier to parse
setTimeout(() => {
console.log("I happen sooner");
setTimeout(() => {
// deeper code
console.log("I happen later");
}, 1);
}, 1);
참고할 만한 글
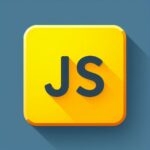
자바스크립트 함수 선언문
이번 포스트에서는 자바스크립트 함수 포스트에서 다루지 못했던 함수 선언문 특징에 대해서 정리해 보았습니다. 함수 선언문의 특징 1 : 호이스팅 함수 선언문은 코드 실행 전에 미리 메모리에 올려지기 때문에 함수를 선언하기 전에 호출할 수 있습니다…. Read more
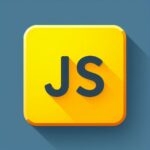
자바스크립트 함수 표현식
이번 포스트에서는 자바스크립트 함수 포스트에서 다루지 못했던 함수 표현식 특징에 대해서 정리해 보았습니다. 함수 표현식 문법 함수 표현식은 다양한 형태로 사용될 수 있습니다. 주의해야 할 점은 function 키워드만 사용하고 이름을 안 넣으면 함수 선언문으로 오해해서 오류가 발생한다는 점입니다…. Read more