들어가며
장고 DTL은 MVT 모델에서 View 단을 떠난 데이터를 template에서 동적으로 다룰 수 있게 해줍니다. 정말 보자마자 너무 유용하다고 느꼈는데요. 이번 포스트에서는 DTL 이란 무엇이고 어떤 태그들이 있는지 정리해보았습니다.
장고 DTL 이란?
Django Template Language (DTL)은 동적 웹 페이지를 구축하기 위한 강력한 도구입니다. 이를 통해 개발자는 Django views에서 제공하는 데이터를 기반으로 HTML로 동적으로 렌더링되는 템플릿을 만들 수 있습니다.
변수, Variable
DTL의 변수는 {{ }} 안에 포함되며 동적 콘텐츠를 출력하는 데 사용됩니다.
{{ variable }}
다음 코드는 user 개체의 first_name 및 last_name 속성에 액세스하여 동적인 인사말을 렌더링해줍니다.
<p>Hello, {{ user.first_name }} {{ user.last_name }}!</p>
템플릿 태그, Template Tags
태그의 기본 형태는 아래와 같습니다.
{% tag %}
Django 템플릿에서 논리 및 제어 흐름을 수행하는 데 사용됩니다.
조건문
{% if user.is_authenticated %}
Welcome back, {{ user.username }}!
{% else %}
Please log in to access this page.
{% endif %}
반복문
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
이 예에서 {% for %} 태그는 items 목록을 반복하고 목록의 각 항목에 대한 목록 항목을 렌더링합니다.
각 하위 리스트의 값을 개별 변수로 압축을 풀 수도 있습니다. 예를 들어 컨텍스트에 점이라는 (x,y) 좌표 목록이 포함되어 있는 경우 다음을 사용하여 점 목록을 출력할 수 있습니다.
{% for x, y in points %}
There is a point at {{ x }},{{ y }}
{% endfor %}
딕셔너리 값도 다음과 같이 반복할 수 있습니다
{% for key, value in data.items %}
{{ key }}: {{ value }}
{% endfor %}
for 태그는 {% empty %}
조건문을 옵션으로 가질 수 있습니다. 해당 조건은 iterable 객체가 empty이거나 찾지 못할 때 수행됩니다.
<ul>
{% for athlete in athlete_list %}
<li>{{ athlete.name }}</li>
{% empty %}
<li>Sorry, no athletes in this list.</li>
{% endfor %}
</ul>
해당 코드는 아래 코드와 동일한 의미입니다.
<ul>
{% if athlete_list %}
{% for athlete in athlete_list %}
<li>{{ athlete.name }}</li>
{% endfor %}
{% else %}
<li>Sorry, no athletes in this list.</li>
{% endif %}
</ul>
include, extend, block
Template를 관리하는데 쓰였던 include, extend, block 또한 결국 template tag의 일종 이였습니다.
복습의 의미로 다시 정리해보면, include
태그는 현재 템플릿 내에 다른 템플릿 파일의 내용을 포함하는 데 사용됩니다.
{% include 'header.html' %}
‘block’ 및 ‘extends’ 태그는 템플릿 상속에 사용되므로 개발자는 공통 요소로 기본 템플릿을 생성하고 하위 템플릿에서 확장할 때 사용됩니다.
url 및 static 태그
url 및 static 태그는 템플릿에서 정적 파일(예: CSS, JavaScript)에 대한 URL 및 참조를 생성하는 데 사용됩니다.
<link rel="stylesheet" href="{% static 'styles.css' %}">
<a href="{% url 'app:index' %}">Home</a>
필터, Filter
필터는 변수의 출력을 수정하거나 형식 지정 작업을 수행하는 데 사용됩니다.
{{ variable|filter }}
date
date
필터는 날짜 및 시간 개체의 형식을 지정하는 데 사용됩니다.
{{ date_value|date:"Y-m-d" }}
views.py
def hello(request):
date = datetime(2024, 6, 15, 10, 25)
return render(request, "hello.html", context={"variable": date})
hello.html
<body>
<h2>환영합니다 유저님!</h2>
<ul>
<li>{{ variable }}</li>
<li>{{ variable|date:"m.d.Y" }}</li>
<li>{{ variable|date:"Y-m-d H:i" }}</li>
</ul>
</body>
브라우저
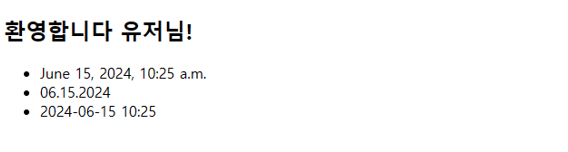
truncatechars
문자열을 지정된 문자 수로 줄입니다.
이 필터는 text 변수를 30자로 자르고 해당 길이를 초과하는 경우 줄임표(…)를 추가해줍니다.
{{ text|truncatechars:30 }}
views.py
def hello(request):
return render(request, "hello.html", context={"text": """고요한 시냇물과 잔잔한 호수 너머,
끝없이 펼쳐진 넓은 바다에는 장엄한 연어가 어슬렁거리고 있습니다.
엄청난 크기와 근육질의 기량을 지닌 연어는 태어날 때부터 민물 강에서
광활한 바다까지 장대한 이동을 시작하고 다시 돌아오는 용감한 수중 세계의 항해자입니다"""})
hello.html
{{ text }}<br><br>
{{ text|truncatechars:100 }}<br><br>
{{ text|truncatechars:30 }}
브라우저
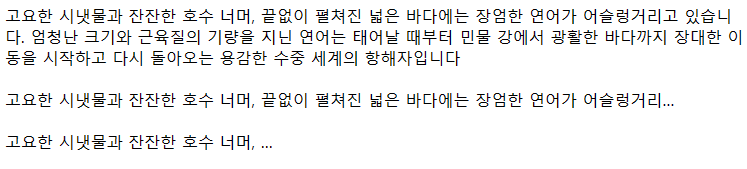
length
문자열 혹은 리스트의 요소 수를 반환합니다.
{{ list|length }}
views.py
def hello(request):
return render(
request, "hello.html",
context={"list": ['사과', '배', '딸기', '키위', '파인애플']})
hello.html
{{ list }} (총 {{ list|length }}개)
브라우저

title
문자열에 있는 각 단어의 첫 글자를 대문자로 표시해줍니다.
{{ title|title}}
views.py
def hello(request):
return render(
request, "hello.html",
context={"title": "Protein secondary structure refers to the local spatial arrangement of \
amino acid residues within a polypeptide chain, \
commonly categorized into alpha helices, beta sheets, and loops."})
hello.html
{{ title }}<br><br>
{{ title|title }}
브라우저
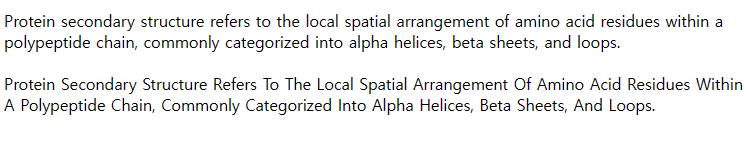
lower and upper
lower
및 upper
필터는 문자열을 각각 소문자 또는 대문자로 변환합니다.
{{ text|lower }}, {{ text|upper }}
views.py
def hello(request):
return render(
request, "hello.html",
context={"text": "Protein secondary structure"})
hello.html
{{ text }}<br><br>
{{ text|upper }}<br><br>
{{ text|lower }}
브라우저
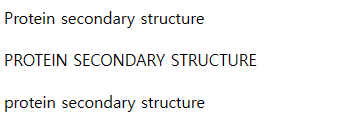
default
default 필터는 변수가 정의되지 않거나 비어 있는 경우 기본값을 설정합니다.
{{ variable|default:"N/A" }}
views.py
def hello(request):
result = []
if result:
answer = f'answer is {result}'
else:
answer = ''
return render(
request, "hello.html",
context={"result": result, "answer": answer})
hello.html
<h3>찾은 결과 표기</h3>
{{ result|default:"None" }}<br><br>
{{ answer|default:"None" }}<br><br>
브라우저
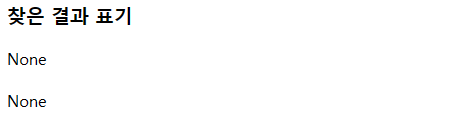
pluralize
pluralize 필터는 개수에 따라 단어에 “s”를 추가합니다.
아래 필터는 count가 2 이상이면 “s”를 뒤에 붙여줍니다.
{{ count }} {{ count|pluralize }}
views.py
def hello(request):
return render(
request, "hello.html",
context={"mail_count": 1, "msg_count": 99, "note_count": 0})
hello.html
<p>
You have {{ mail_count }} mail{{ mail_count|pluralize }}
</p>
<p>
You have {{ msg_count }} message{{ msg_count|pluralize }}
</p>
<p>
You have {{ note_count }} note{{ note_count|pluralize }}
<!-- 0이하는 단수로 인식하지만 0은 복수로 인식함에 유의 -->
</p>
브라우저
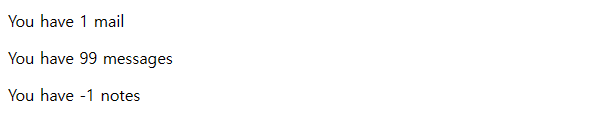
filter
파이프를 사용하여 하나 이상의 필터를 통해 블록의 내용을 필터링합니다.
filter 와 endfilter 태그 사이의 모든 텍스트를 대상으로 filter가 적용됩니다.
views.py
def hello(request):
return render(
request, "hello.html",
context={"title": "Protein secondary structure refers to the local spatial arrangement of \
amino acid residues within a polypeptide chain, \
commonly categorized into alpha helices, beta sheets, and loops."})
hello.html
{{ title }}<br><br>
{% filter title|truncatechars:70 %}
{{ title }}
{% endfilter %}
브라우저

firstof
“false”가 아닌(즉, 존재하고, 비어 있지 않으며, false 부울 값이 아니고, 0 숫자 값이 아닌) 첫 번째 인수 변수를 출력합니다. 전달된 변수가 모두 “false”인 경우 아무것도 출력하지 않습니다.
views.py
def hello(request):
return render(
request, "hello.html",
context={"var1": [], "var2": [2, 3], "var3": [100]})
hello.html
{% firstof var1 var2 var3 "fallback value" %}
브라우저
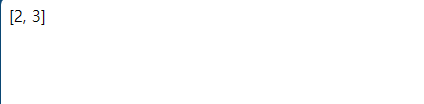
주석, Comments
DTL의 주석은 {# #}
안에 포함되며 템플릿 렌더링 중에 무시됩니다.
{# 한 줄 주석 #}
{% comment %}
여러줄
주석
{% endcomment %}
그런데 DTL도 HTML위에서 작동하는데 HTML에는 이미 주석 코드가 있다는 것을 아실 겁니다.
<!-- This is an HTML comment -->
둘의 차이점은 HTML 주석은 렌더링된 웹 페이지의 소스 코드에 표시되지만 DTL 주석은 템플릿 렌더링 중에 제거되므로 표시되지 않는다는 점입니다.
마치며
재밌는 DTL들이 너무 많습니다. 하지마 너무 딥 다이브 하기에는 장고 공부가 우선이라, 오늘은 여기까지만 공부해보았습니다.
차후에 추가로 공부하거나 필요에 의해 알게 된 DTL 들이 있다면 따로 정리 해보도록 하겠습니다.
참고하면 좋은 글
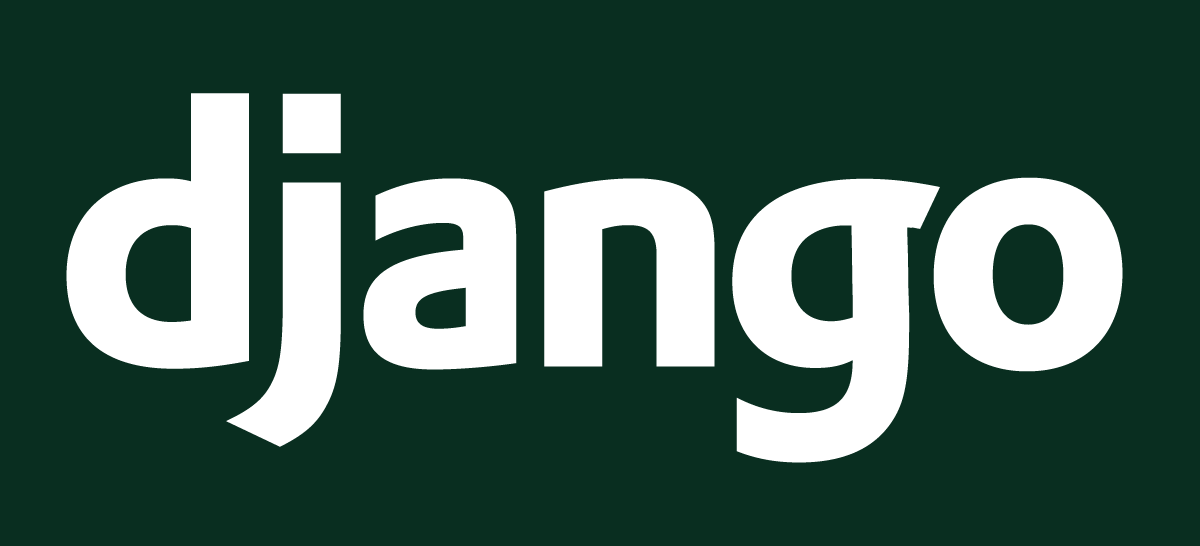
Built-in template tags and filters | Django documentation | Django (djangoproject.com)
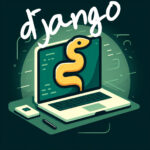
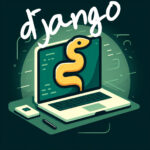
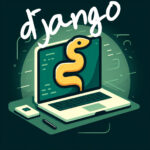